Pinterest Scraper Simplified: From No-Code to Coding Pinterest Scraping Techniques
Pinterest is not just a place for finding recipes or DIY ideas; it's also full of opportunities for businesses.
As Pinterest CEO Ben Silbermann puts it,
“The whole reason Pinterest exists is to help people discover the things that they love and then go take action on them, and a lot of the things they take action on are tied to commercial intent.”
Last year, Pinterest’s Monthly Active Users crossed the limits and jumped up to a whopping 498 million users! That's a lot of people and a lot of potential for you to scrape Pinterest for commercial or personal benefits.
This blog shows you how to scrape Pinterest through a user-friendly Pinterest Scraper and a more technical way using Python.
So, whether you're new to this or already know a bit about coding, we've got you covered. But before we begin, let’s learn about Pinterest’s stance on scraping.
Is it Legal to Scrape Pinterest?
Pinterest Terms of Service explicitly state that scraping Pinterest data and collecting it using automated means is prohibited. This includes collecting data through any form of Pinterest scraper or coding scripts.
But Pinterest has an official API, right? How about scraping Pinterest using the Pinterest API? That, too, is forbidden. Pinterest makes it clear in its developer guidelines that data extraction from its platform is an unacceptable use of Pinterest API and other developer tools.
However, a general rule of thumb for most platforms is that scraping is permitted as long as the data is publicly available and doesn’t require a login. You should also avoid scraping copyrighted and personal content, as doing so can have legal repercussions.
In short, as long as your approach to using a Pinterest scraper is harmless and your use of extracted data is legal, you’re in safe waters.
Pinterest Scraper With and Without Code
Now that we understand the legal implications of scraping Pinterest let's show you how to use a no-code Pinterest scraper and also build a Pinterest scraper using Python.
No-Code Pinterest Scraper
Scraping Pinterest for data can seem daunting, especially if you're not well-versed in coding. Thankfully, no-code tools have made web scraping Pinterest not only possible but remarkably easy.
Apify, Octoparse, and ParseHub are among the top Pinterest scrapers for their efficiency and user-friendly interface.
For this guide, we’ll be using the Apify Pinterest Scraper.
The Apify Pinterest bot turns the complexity of data extraction into a simple, manageable process. This tool is designed to seamlessly navigate Pinterest and extract pins, boards, and user profile information without a hitch.
Here’s a concise guide on how to scrape Pinterest using Apify Pinterest Scraper without any hassle.
Step 1: Visit the Apify Store’s Pinterest Scraper Page
Head over to the Apify Store and find the Pinterest Scraper tool. Hit the “Try for free” button.
Step 2: Set up an Account:
Pressing the “Try for Free” button will land you on the login/signup page. Sign up on Apify using your email address and a secure password. Or simply use your Google or GitHub account to create an account.
If you’re already part of the Apify family, skip ahead to step 3.
After successful signup, you’ll be taken to the online Apify Console. Yes, no browser extension or software package to download!
Step 3: Choose Your Pinterest Data Source
In the Apify Console, you’ll find a field asking for the URL of the Pinterest profile or pins you’re interested in. Paste your target Pinterest profiles here.
You can also post them all at once by clicking the Bulk Edit button.
Step 4: Kickstart the Scraping Process
Once you are done entering all the target URLs and usernames, hit the Save & Start button and let the Pinterest Scraper do its thing.
You’ll know it’s done when the status flips from Running to Succeeded.
Step 5: Collect Your Data
Once the job’s done, hop over to the Storage tab.
Here, you’ll find your freshly scraped Pinterest data ready for review. You can check it out in various formats like HTML, JSON, CSV, Excel, XML, and even RSS feed.
You have the option to select specific fields and omit irrelevant fields before downloading the dataset. Once you are done with that, download it in your preferred format, and voilà, the data is at your disposal.
Scrape Pinterest using Python
Scraping Pinterest using Python can be a straightforward process with the right tools and a bit of coding knowledge.
Here's a concise guide to creating your own Pinterest scraper Python script using the requests and BeautifulSoup libraries. The guide provides a surface-level explanation of the process, perfect for beginners or those looking for a quick overview.
Step 1: Set Up Your Environment
Ensure you have Python installed on your computer. You'll also need the requests and BeautifulSoup libraries, which can be installed via pip if you haven't already.
Step 2: Write Your Scraper Class
Begin by importing the necessary modules:
-
requests for making HTTP requests to Pinterest and
-
BeautifulSoup from bs4 for parsing the HTML content
Step 3: Loading the Images
Create a method in your class (load_images) to read the HTML content of the page where Pinterest images are listed. This method reads from a locally saved HTML file (images.html), which you should have saved beforehand from Pinterest.
Step 4: Parsing the Images
Implement another method (parse) that takes the HTML content as input and uses BeautifulSoup to parse it. Extract the src attributes of all <img> tags to get the URLs of the images you want to download.
Step 5: Downloading the Images
Write a download method that takes an image URL, fetches the image using the requests.get method, and saves it locally. Ensure you handle the response correctly, checking for a successful status code (200) before proceeding with saving the file.
Step 6: Running the Scraper
Define a run method that ties everything together: load the HTML content, parse it to extract image URLs, and then download each image.
Step 7: Execute Your Script
With your PinterestScraper class defined, make sure to invoke the run method within a if __name__ == '__main__': block to start the scraping process when you execute the script.
Combining all the snippets above gives us the complete script for scraping Pinterest:
This is a simplified guide and gives you a basic framework for a Pinterest scraper Python script.
Scrape Pinterest Without Getting Blocked
Pinterest strictly prohibits unauthorized data collection using automated means without explicit permission. Pinterest may suspend your account or block your IP upon sensing scraping activities from your end.
This creates a challenge for those needing to scrape Pinterest ethically for legal purposes.
But fret not. AdsPower has a solution for you. AdsPower antidetect browser is tailored for web scraping Pinterest. It makes your Pinterest scraper mimic human activity, thus reducing the risk of detection.
It uses IP rotation and browser fingerprinting techniques to make your Pinterest scraper more discreet and efficient. The tool is also equipped with helpful features for automating the scraping process and further reducing the workload.
So, before you start scraping Pinterest, make sure that AdsPower is onboard for secure and seamless scraping.
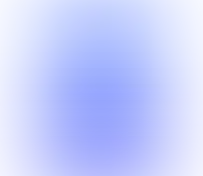
People Also Read
- WhatsApp Web Login: A Comprehensive Guide to Using WhatsApp Web
- Where To Promote Affiliate Links? 6 Best and Most Used Channels
- Navigating Google Ads Agency Accounts: A Comprehensive Guide
- 5 Best No KYC Crypto Exchange and Why You Should Use Them
- How AdsPower Antidetect Browser Works for Digital Agencies & Media Buyers